Install
Install the package:
npm add @dmno/encrypted-vault-plugin
pnpm add @dmno/encrypted-vault-plugin
yarn add @dmno/encrypted-vault-plugin
bun add @dmno/encrypted-vault-plugin
Initialize the plugin
Initialize the plugin in the root, or service if not shared. Note the vault/prod
id, which we can refer to in other services or in the CLI. This is useful if you have multiple vaults.
Also, note the configPath
function. This is a helper to wire up the encryption key plugin input to the location of that value in your service’s config. We obviously don’t want to hardcode that key in this file, so this lets it live within the rest of our config, and pull in the value from a .env.local
file or environment variable. The plugin has it’s own internal config schema, so the first argument of '..'
tells us to look in the plugin’s parent - the service.
import { defineDmnoService, configPath } from 'dmno';import { EncryptedVaultDmnoPlugin, EncryptedVaultTypes } from '@dmno/encrypted-vault-plugin';
const MyProdVault = new EncryptedVaultDmnoPlugin('vault/prod', { key: configPath('..', 'DMNO_VAULT_KEY'),});
export default defineDmnoService({ schema: { DMNO_VAULT_KEY: { extends: EncryptedVaultTypes.encryptionKey, // NOTE - the type itself is already marked as secret }, },});
If your plugin was initiatized in root and you need to use in a child service, inject the already configured plugin:
import { EncryptedVaultDmnoPlugin } from '@dmno/encrypted-vault-plugin';
const MyVault = EncryptedVaultDmnoPlugin.injectInstance('vault/prod'); // same "instance name" it was created with
Initialize the vault and key
npm exec -- dmno plugin -p vault/prod -- setup
pnpm exec dmno plugin -p vault/prod -- setup
yarn exec -- dmno plugin -p vault/prod -- setup
bun run dmno plugin -p vault/prod -- setup
This will:
- detect if the vault is configured but has no key value
- detect if vault file is empty/exists
- create new a key if needed
Add vault items to your schema
{ // simple case example SUPER_SECRET_ITEM: { value: MyProdVault.item(), }, ITEM_WITH_PROD_ONLY_SECRET: { value: toggleByNodeEnv({ _default: 'not-a-secret', staging: NonProdVault.item(), // reference to another vault production: MyProdVault.item(), }) },}
Fill the vault with your secrets
Add encrypted values to the vault:
npm exec -- dmno plugin -p vault -- add
pnpm exec dmno plugin -p vault -- add
yarn exec -- dmno plugin -p vault -- add
bun run dmno plugin -p vault -- add
Rotate the vault key
npm exec -- dmno plugin -p vault -- rotate-key
pnpm exec dmno plugin -p vault -- rotate-key
yarn exec -- dmno plugin -p vault -- rotate-key
bun run dmno plugin -p vault -- rotate-key
This will:
- generate a new key, and share it, similar to the initial setup
- re-encrypts all the values in the vault with the new key
Accessing an existing vault
If you’re joining a project that already has a vault set up, you’ll will need to get the key from a coworker.
Plugin CLI reference
Reference
Description: Runs CLI commands related to a specific plugin instance
Options
-s, --service [service]
which service to load
-p, --plugin <plugin>
which plugin instance to interact with
Example(s)
# set up a new encrypted vaultdmno plugin -p vault -- setup
# Update or insert an item to te vaultdmno plugin -p vault -- upsert
# add an item to the vaultdmno plugin -p vault -- add
# update an item in the vaultdmno plugin -p vault -- update
# delete an item from the vaultdmno plugin -p vault -- delete
# delete an item from the vaultdmno plugin -p vault -- delete
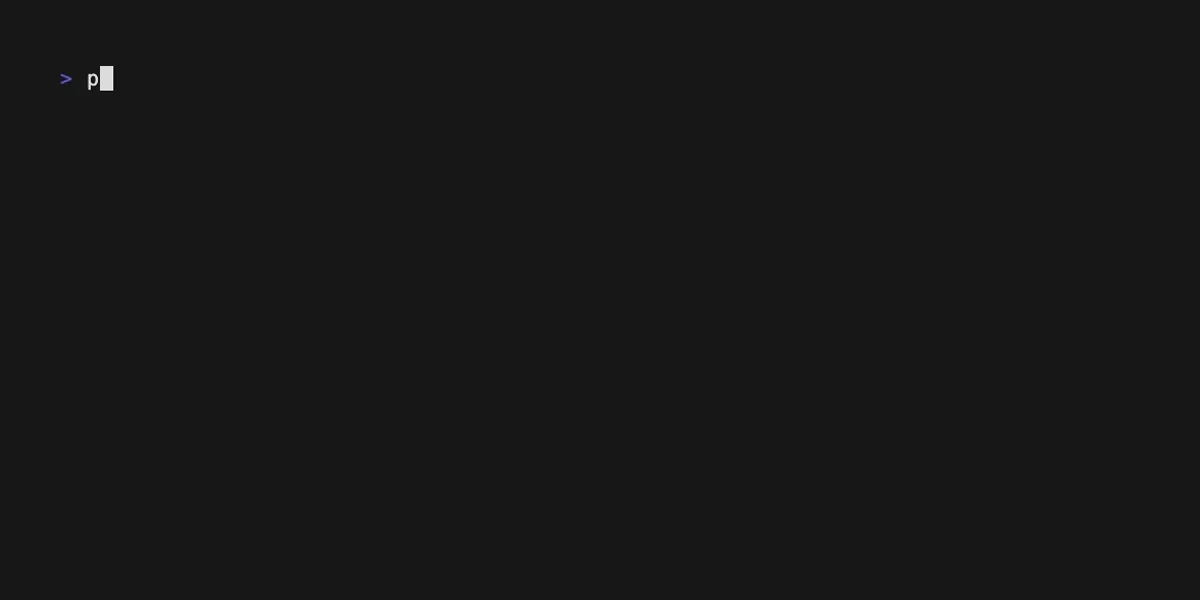